一、多线程List集合不安全
1.1、List集合不安全案例代码
/**
* @Author : 一叶浮萍归大海
* @Date: 2023/11/20 12:38
* @Description: 多线层环境下List集合不安全案例代码
*/
public class NotSafeListMainApp {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
for (int i = 1; i <= 30; i++) {
new Thread(() -> {
list.add(UUID.randomUUID().toString().toLowerCase().substring(0, 8).replaceAll("-", ""));
System.out.println("【当前线程】:" + Thread.currentThread().getName() + ",【当前list】:" + list);
}, String.valueOf(i)).start();
}
}
}
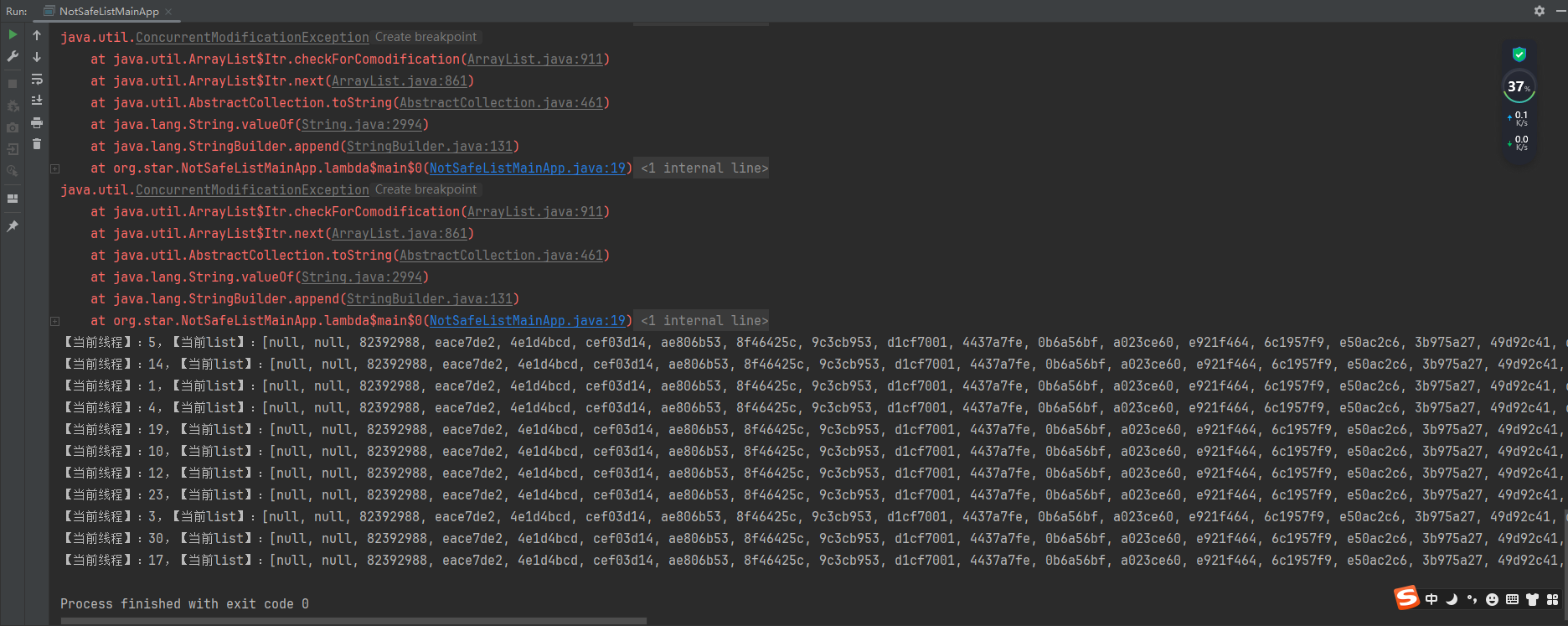
1.2、解决方法
/**
* @Author : 一叶浮萍归大海
* @Date: 2023/11/20 12:38
* @Description: 多线层环境下List集合不安全解决案例代码
*/
public class NotSafeListPlusMainApp {
public static void main(String[] args) {
// List<String> list = Collections.synchronizedList(new ArrayList<>());
List<String> list = new CopyOnWriteArrayList<>();
for (int i = 1; i <= 30; i++) {
new Thread(() -> {
list.add(UUID.randomUUID().toString().toLowerCase().substring(0, 8).replaceAll("-", ""));
System.out.println("【当前线程】:" + Thread.currentThread().getName() + ",【当前list】:" + list);
}, String.valueOf(i)).start();
}
}
}
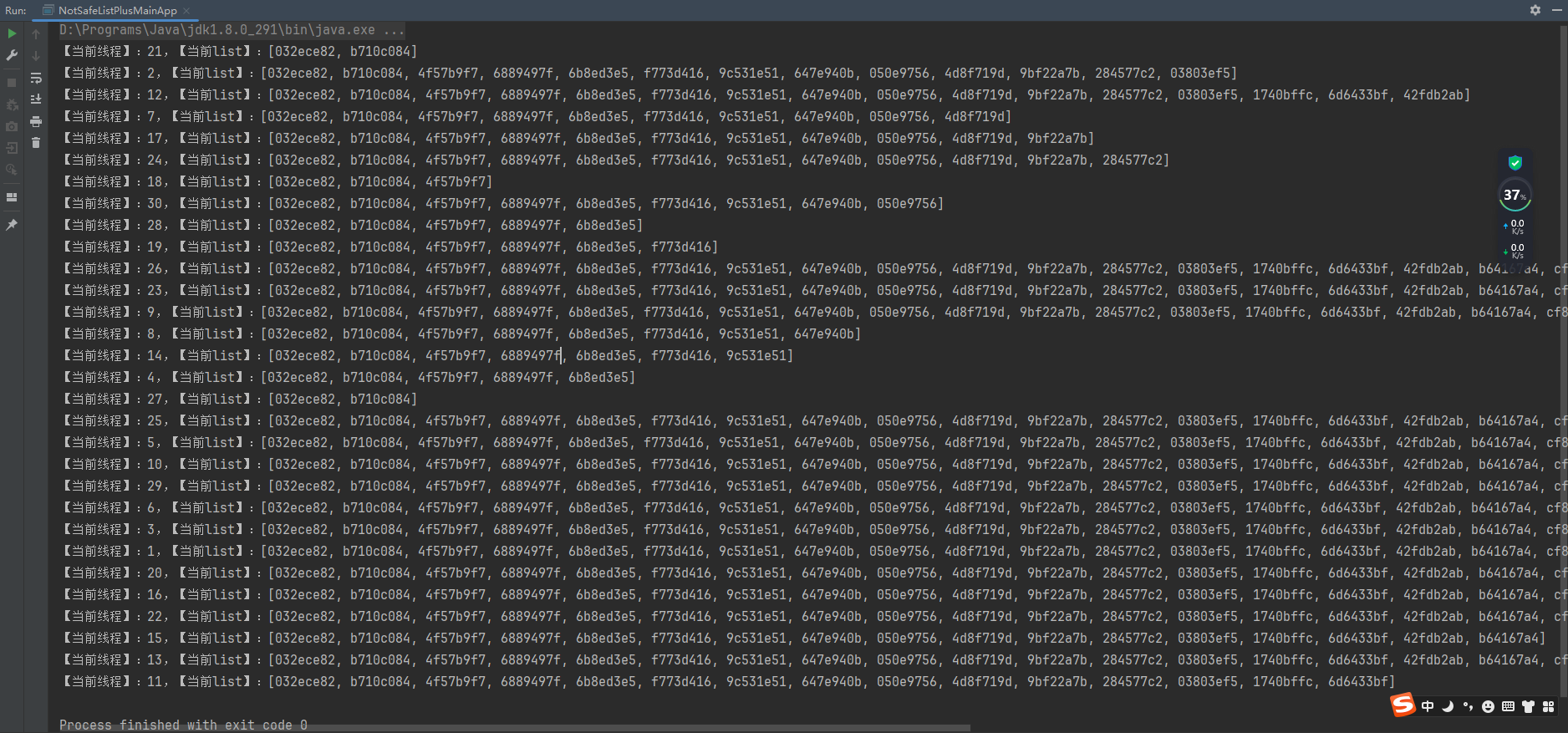
二、多线程HashSet集合不安全
2.1、HashSet集合不安全案例代码
/**
* @Author : 一叶浮萍归大海
* @Date: 2023/11/20 12:38
* @Description: 多线层环境下HashSet集合不安全案例代码
*/
public class NotSafeHashSetMainApp {
public static void main(String[] args) {
Set<String> hashSet = new HashSet<>();
for (int i = 1; i <= 30; i++) {
new Thread(() -> {
hashSet.add(UUID.randomUUID().toString().toLowerCase().substring(0, 8).replaceAll("-", ""));
System.out.println("【当前线程】:" + Thread.currentThread().getName() + ",【当前hashSet】:" + hashSet);
}, String.valueOf(i)).start();
}
}
}
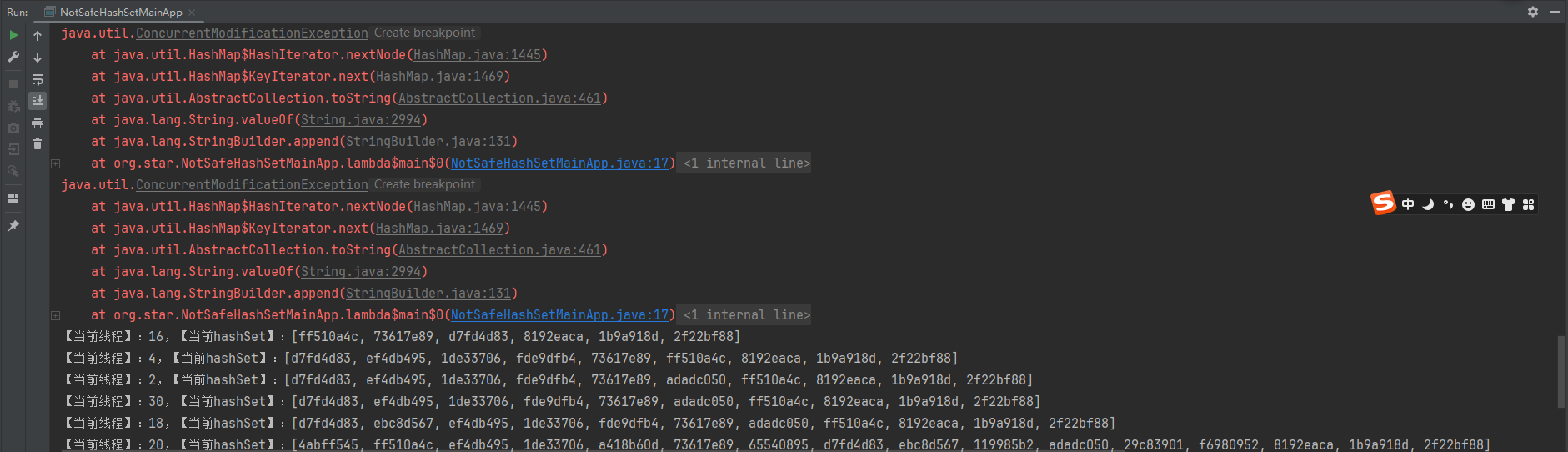
2.2、解决方法
/**
* @Author : 一叶浮萍归大海
* @Date: 2023/11/20 12:38
* @Description: 多线层环境下HashSet集合不安全解决案例代码
*/
public class NotSafeHashSetPlusMainApp {
public static void main(String[] args) {
// Set<String> hashSet = Collections.synchronizedSet(new HashSet<>());
Set<String> hashSet = new CopyOnWriteArraySet<>();
for (int i = 1; i <= 30; i++) {
new Thread(() -> {
hashSet.add(UUID.randomUUID().toString().toLowerCase().substring(0, 8).replaceAll("-", ""));
System.out.println("【当前线程】:" + Thread.currentThread().getName() + ",【当前hashSet】:" + hashSet);
}, String.valueOf(i)).start();
}
}
}
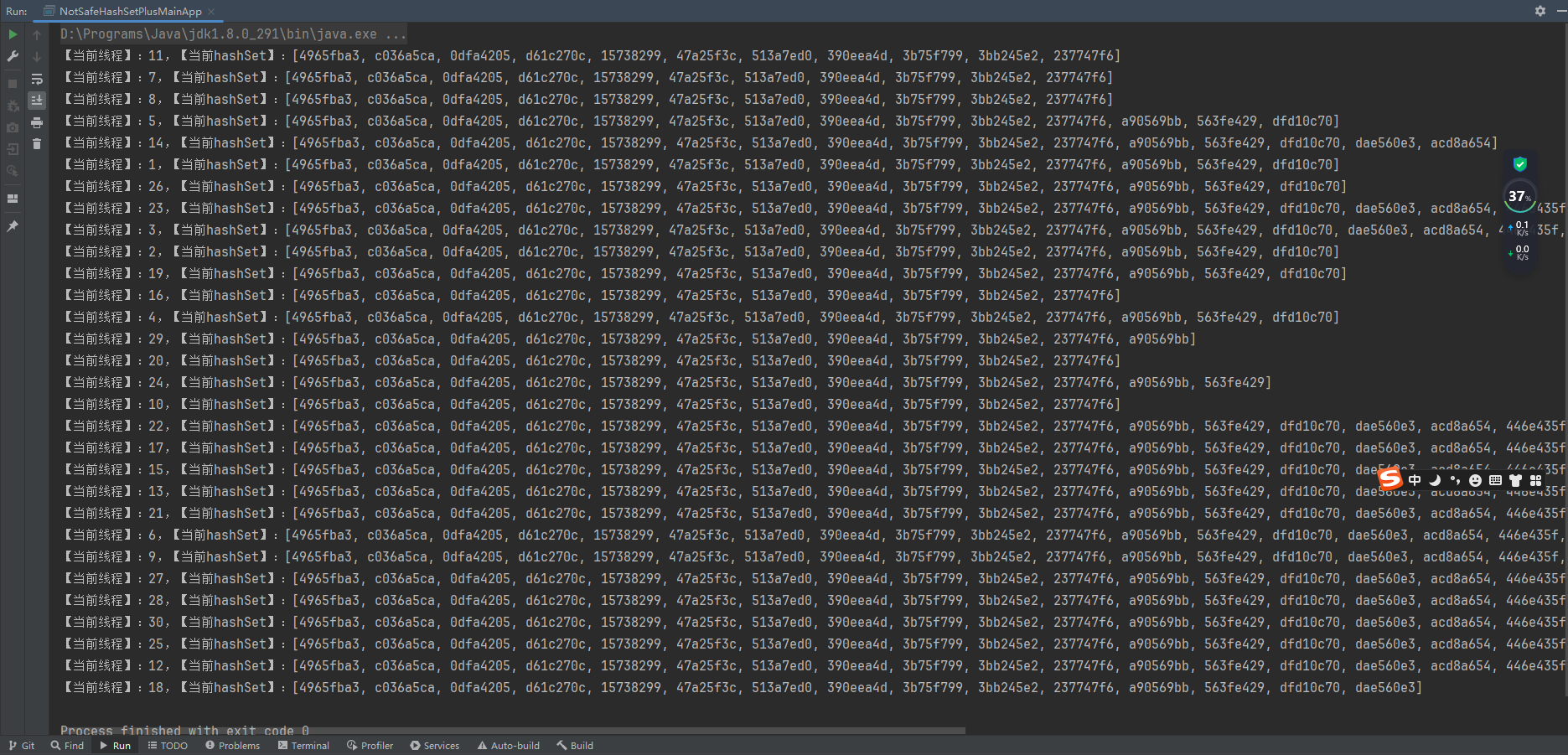
三、多线程HashMap集合不安全
3.1、HashMap集合不安全案例代码
/**
* @Author : 一叶浮萍归大海
* @Date: 2023/11/20 12:38
* @Description: 多线层环境下HashMap集合不安全案例代码
*/
public class NotSafeHashMapMainApp {
public static void main(String[] args) {
HashMap<String,Object> hashMap = new HashMap<>();
for (int i = 1; i <= 30; i++) {
new Thread(() -> {
hashMap.put(UUID.randomUUID().toString().substring(0, 1),UUID.randomUUID().toString().toLowerCase().substring(0, 8).replaceAll("-", ""));
System.out.println("【当前线程】:" + Thread.currentThread().getName() + ",【当前hashMap】:" + hashMap);
}, String.valueOf(i)).start();
}
}
}
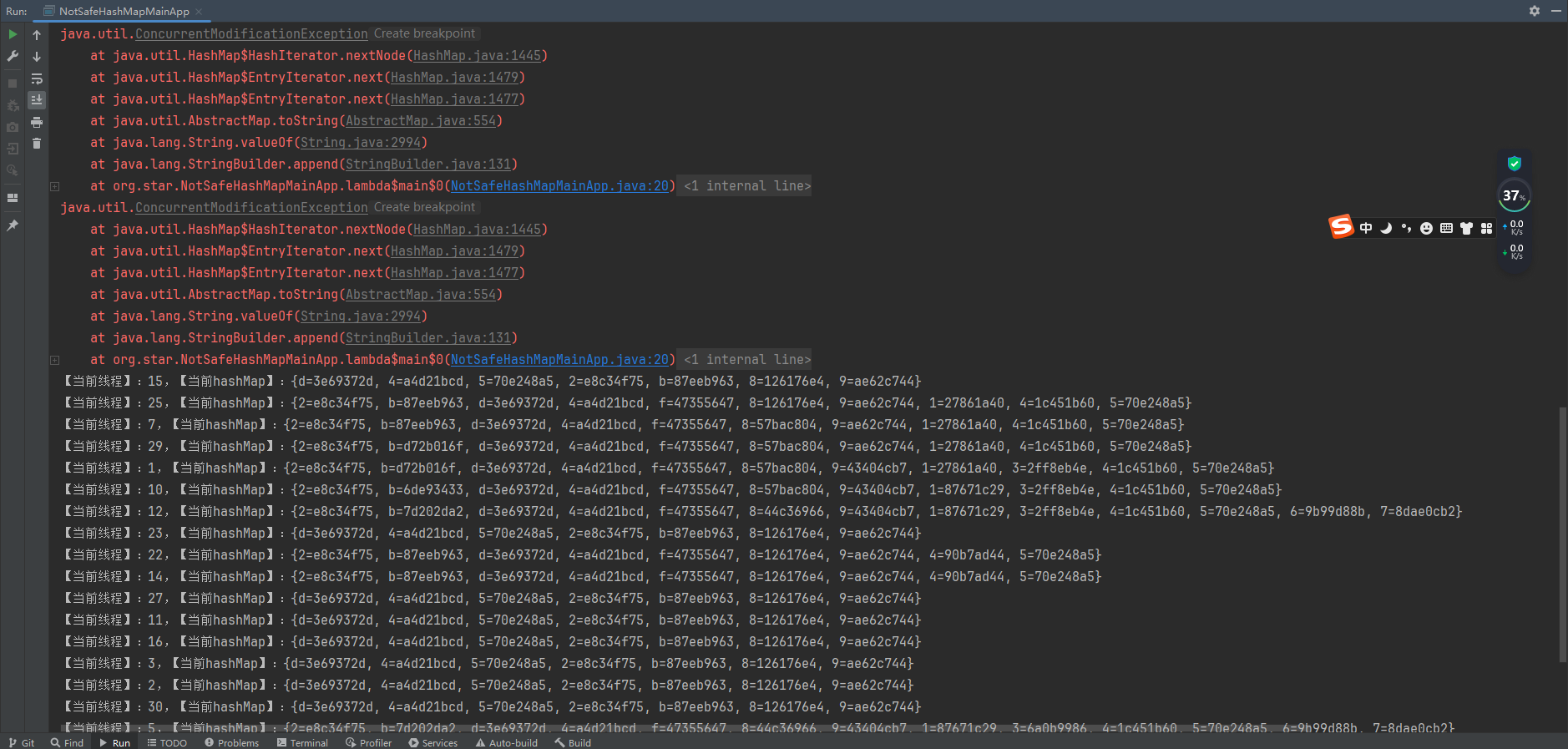
3.2、解决方法
/**
* @Author : 一叶浮萍归大海
* @Date: 2023/11/20 12:38
* @Description: 多线层环境下HashMap集合不安全解决案例代码
*/
public class NotSafeHashMapPlusMainApp {
public static void main(String[] args) {
// Map<String,Object> hashMap = Collections.synchronizedMap(new HashMap<>());
Map<String,Object> hashMap = new ConcurrentHashMap<>();
for (int i = 1; i <= 30; i++) {
new Thread(() -> {
hashMap.put(UUID.randomUUID().toString().substring(0, 1),UUID.randomUUID().toString().toLowerCase().substring(0, 8).replaceAll("-", ""));
System.out.println("【当前线程】:" + Thread.currentThread().getName() + ",【当前hashMap】:" + hashMap);
}, String.valueOf(i)).start();
}
}
}
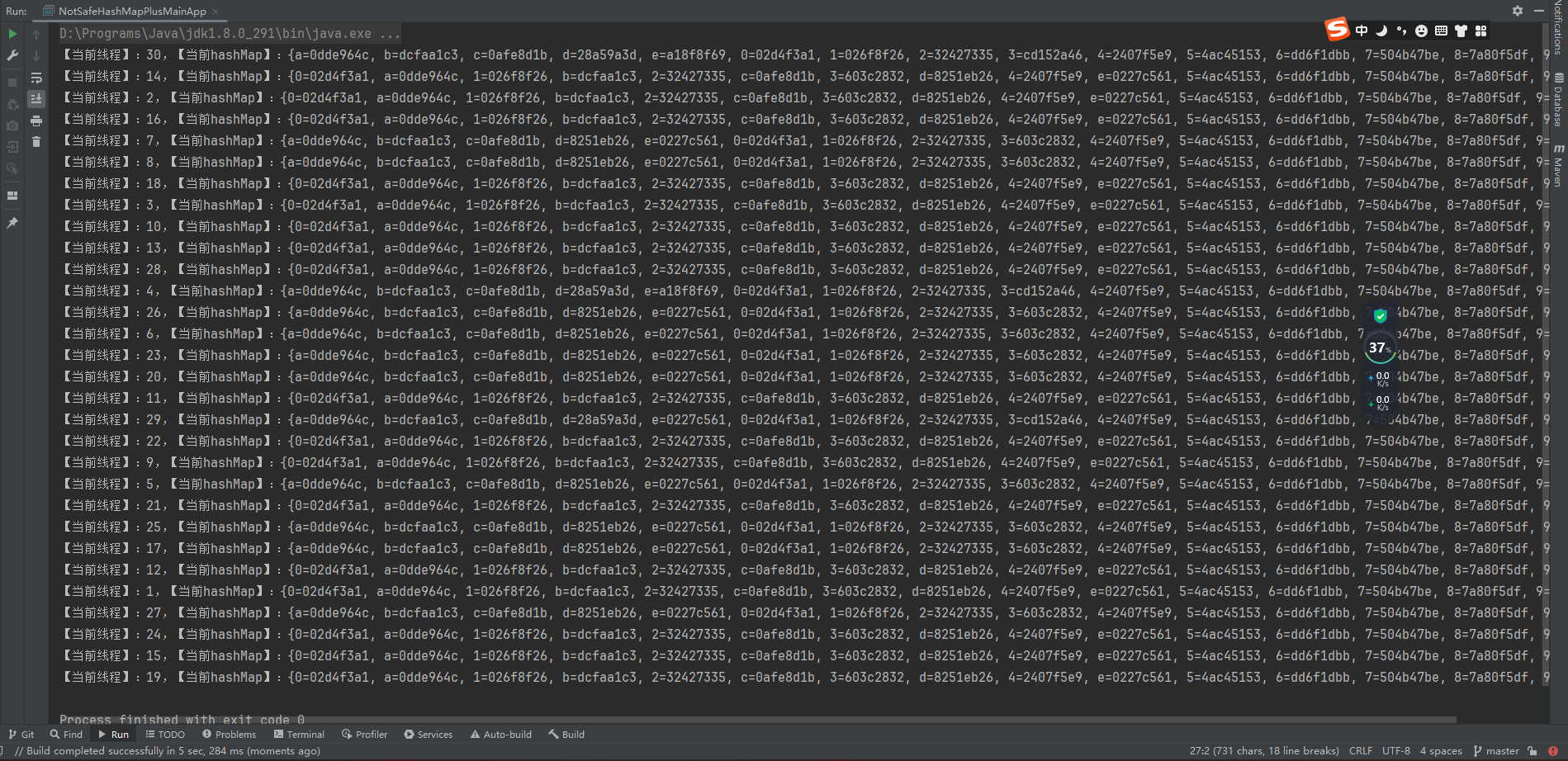